1. Batch writing
한 번에 많은 데이터를 로드하는 경우 DynamoDb.Table.batch_writer()를 사용하여 프로세스 속도를 높이고 서비스에 대한 쓰기 요청 수를 줄일 수 있다.
# batch_put_item.py
import boto3
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('users')
with table.batch_writer() as batch:
batch.put_item(
Item={
'account_type': 'standard_user',
'username': 'johndoe',
'first_name': 'John',
'last_name': 'Doe',
'age': 25,
'address': {
'road': '1 Jefferson Street',
'city': 'Los Angeles',
'state': 'CA',
'zipcode': 90001
}
}
)
batch.put_item(
Item={
'account_type': 'super_user',
'username': 'janedoering',
'first_name': 'Jane',
'last_name': 'Doering',
'age': 40,
'address': {
'road': '2 Washington Avenue',
'city': 'Seattle',
'state': 'WA',
'zipcode': 98109
}
}
)
batch.put_item(
Item={
'account_type': 'standard_user',
'username': 'bobsmith',
'first_name': 'Bob',
'last_name': 'Smith',
'age': 18,
'address': {
'road': '3 Madison Lane',
'city': 'Louisville',
'state': 'KY',
'zipcode': 40213
}
}
)
batch.put_item(
Item={
'account_type': 'super_user',
'username': 'alicedoe',
'first_name': 'Alice',
'last_name': 'Doe',
'age': 27,
'address': {
'road': '1 Jefferson Street',
'city': 'Los Angeles',
'state': 'CA',
'zipcode': 90001
}
}
)
위 코드에서 보다시피 데이터의 양이 매우 많을 때는 batch.put_item 사용이 권장된다.
E:\boto3>python batch_put_item.py
해당 코드를 cmd창에서 실행해준다.
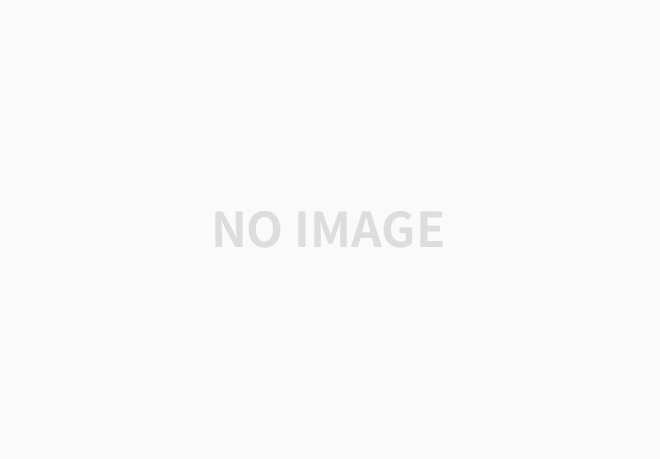
AWS 콘솔에 접속하여 확인하면 위와 같이 입력한 데이터가 테이블에 등록되어있다.
2. For문을 사용한 Batch writing
# batch_put_item2.py
import boto3
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('users')
with table.batch_writer() as batch:
for i in range(50):
batch.put_item(
Item={
'account_type': 'anonymous',
'username': 'user' + str(i),
'first_name': 'unknown',
'last_name': 'unknown'
}
)
위와 같이 for문을 사용하여 매우 많은 양의 데이터를 넣을 때에도 사용된다.
E:\boto3>python batch_put_item2.py
해당 코드를 cmd창에서 실행해준다.
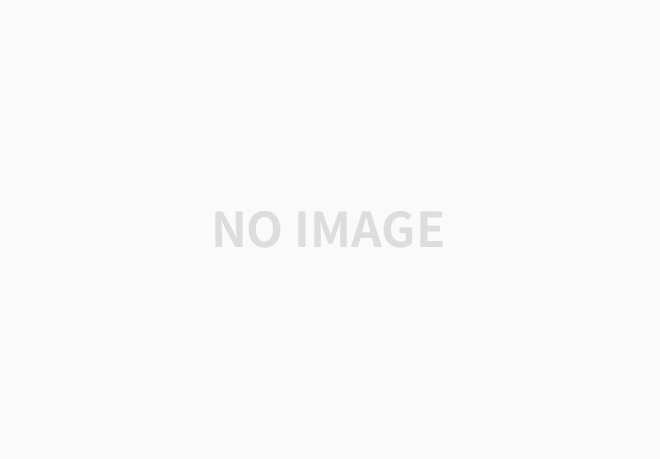
수 많은 데이터가 매우 빠른속도로 테이블에 입력된다.
3. 기본키를 사용한 데이터 입력 예제
# batch_put_item3.py
import boto3
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('users')
with table.batch_writer(overwrite_by_pkeys=['username', 'last_name']) as batch:
batch.put_item(
Item={
'username': 'p1',
'last_name': 's1',
'other': '111',
}
)
batch.put_item(
Item={
'username': 'p1',
'last_name': 's1',
'other': '222',
}
)
batch.delete_item(
Key={
'username': 'p1',
'last_name': 's2'
}
)
batch.put_item(
Item={
'username': 'p1',
'last_name': 's2',
'other': '444',
}
)
overwrite_by_pkeys=['partition_key', 'sort_key'] 구문이 추가되어 작성된 것을 볼 수 있다.
pkeys는 기본키가 들어가며, 파티션 키, 정렬 키의 값이 들어간다.
위 예제 코드는 [p1,s1,111], [p1,s1,222] 값을 테이블에 입력한 후 [p1,s2] 키의 값을 삭제하고 다시 [p1,s2,444] 값을 입력하도록 작성되어있다.
기본 키가 같을 경우 세부 속성이 달라도 같은 값이 들어가면 안되기 때문에 위의 코드는 오류가 발생해야한다.
E:\boto3>python batch_put_item3.py
하지만 해당 코드를 cmd창에서 실행하면 정상작동한다.
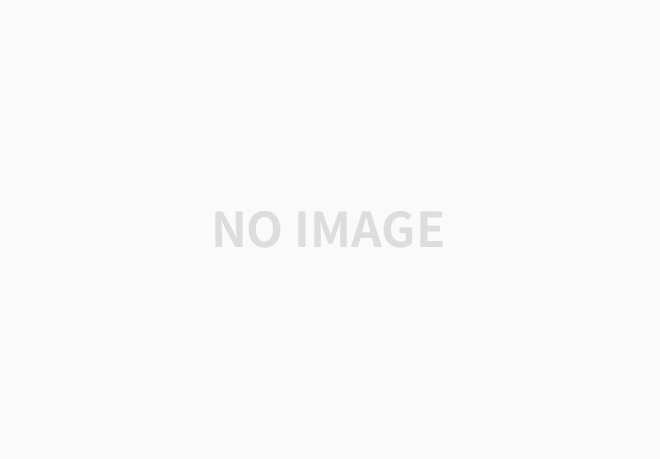
[p1,s1,222], [p1,s2,444] 이 두개의 데이터만 들어간 것을 볼 수 있다.
이는 overwrite by pkeys를 작성했기 때문에 오류가 발생하지 않는 것이다.
만약 동일한 기본키 값이 들어오게 되면 기존의 item에 덮어 씌우는 식으로 작동한다.
즉 [p1,s1,111]이 먼저 입력되지만 이후에 [p1,s1,222]가 overwrite되어 기존의 item은 삭제되었다고 보면 된다.
import boto3
dynamodb = boto3.resource('dynamodb')
table = dynamodb.Table('users')
batch.put_item(
Item={
'partition_key': 'p1',
'sort_key': 's1',
'other': '222',
}
)
batch.put_item(
Item={
'partition_key': 'p1',
'sort_key': 's2',
'other': '444',
}
)
위 코드의 중복을 제거하여 overwrite 기능을 사용하지 않고 진행하려면 위와 같은 코드가 작성되어야 한다.
'AWS CLI, Boto3' 카테고리의 다른 글
DynamoDB with Boto3(Python) 4편 (0) | 2021.07.18 |
---|---|
DynamoDB with Boto3(Python) 2편 (0) | 2021.07.16 |
DynamoDB with Boto3(Python) 1편 (0) | 2021.07.16 |
Boto3를 활용한 S3 bucket 관리 (0) | 2021.07.15 |
boto3 설치와 설정 및 간단하게 사용해보기 (0) | 2021.07.14 |